일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 | 31 |
- yolo
- computervision
- Text To Speech
- pypdf2
- processstart
- 사무자동화 #Selenium
- pythonnet
- Text-to-Speech
- pyautogui
- pdf merge
- objectdetection
- YOLOv7
- DeepLearning
- YOLOv5
- Google API
- 파이썬 #업무자동화 #python
- google cloud
- ironpython
- 사무자동화
- 업무자동화
- Today
- Total
Doarchive
[OpenCV] 허프 변환을 이용한 원 검출 Hough Circle Transform 본문
[OpenCV] 허프 변환을 이용한 원 검출 Hough Circle Transform
오순발닦개 2022. 12. 2. 22:01cv2.HoughCircles(image: Mat, method: int, dp, minDist, param1 param2, minRadius, maxRadius)
image = 8-bit, single-channel, grayscale input image
method = 검출방식 #HOUGH_GRADIENT / #HOUGH_GRADIENT_ALT.
dp= 입력 영상과 경사 누적의 해상도 반비례율, 1: 입력과 동일, 값이 커질수록 부정확
dp=1 , the accumulator has the same resolution as the input image.
dp=2 , the accumulator has half as big width and height.
HOUGH_GRADIENT_ALT : recommended value =1.5, (아주 작은 원을 검출할 경우 제외)
minDist = 원들 중심 간의 최소 거리 (0이면 안됨, 동심원 검출 불가)
매개 변수가 너무 작으면 실제원 외에 잘못 검출할 수 있고 , 너무 크면 일부 원을 놓칠 수 있음.
param1 Canny Edge Detector 에 사용되는 파라메터 두개중 높은 임계값입니다( 임계값은 이 파라메터의 절반)
#HOUGH_GRADIENT_ALT threshold value normally be higher, such as 300 or normally exposed and contrasty images.
param2 경사도 누적 경계 값 (값이 작을수록 잘못된 원 검출 , 클수록 검출률이 낮아짐)
#HOUGH_GRADIENT, it is the accumulator threshold for the circle centers at the detection stage.
The smaller it is, the more false circles may be detected.
Circles, corresponding to the larger accumulator values, will be returned first.
#HOUGH_GRADIENT_ALT algorithm, this is the circle "perfectness" measure.
The closer it to 1, the better shaped circles algorithm selects. In most cases 0.9 should be fine.
If you want get better detection of small circles, you may decrease it to 0.85, 0.8 or even less.
But then also try to limit the search range [minRadius, maxRadius] to avoid many false circles.
minRadius / maxRadius 원의 최소 반지름, 최대 반지름 (0이면 이미지 전체의 크기)
If maxRadius <= 0, uses the maximum image dimension.
If maxRadius < 0, #HOUGH_GRADIENT returns centers without finding the radius.
#HOUGH_GRADIENT_ALT always computes circle radiuses.
return = circles(optional): 검출 원 결과, N x 1 x 3 부동 소수점 배열 (x, y, 반지름)
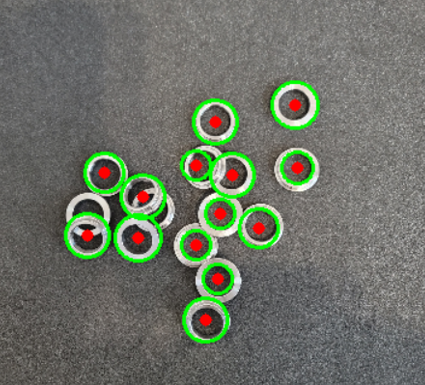
Hough gradient method
가장자리에 그레이디언트 방법을 이용해 원의 중심점(a, b)에 대한 2차원 히스토그램을 선정
모든 점에 대해 최소 거리에서 최대 거리까지 기울기의 선분을 따라 누산 평면의 모든 점을 증가시킴
중심점을 선택 -> 중심점 후보군에서 임곗값보다 크고 인접한 점보다 큰 점을 중심점으로 선택
선정된 중심점(a, b)와 가장자리의 좌표를 원의 방정식에 대입해 반지름 r의 1차원 히스토그램으로 판단
2차원 평면 공간에서 축적 영상을 생성->엣지에서 그래디언트 계산->에지 방향으로 직선을 그리면서 값을 누적 -> 원의 중심찾은 뒤 반지름을 검출
트랙바를 이용해 HoughCircles 의 적절한 파라미터를 찾는 예제
import cv2
import numpy as np
filePath='D:/Project/Python/Resource/Image/circle3.jpg'
image = cv2.imread(filePath,cv2.IMREAD_COLOR)
image =cv2.resize(image,(400,400))
# 그레이 스케일 변환
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 노이즈 제거를 위한 가우시안 블러
blur = cv2.GaussianBlur(gray, (3,3), 0)
def on_trackbar(pos):
minDist=cv2.getTrackbarPos('minDist','img')
cmax=cv2.getTrackbarPos('canny_max','img')
thres=cv2.getTrackbarPos('thres','img')
minRadius=cv2.getTrackbarPos('minRadius','img')
maxRadius=cv2.getTrackbarPos('maxRadius','img')
circles = cv2.HoughCircles(blur, cv2.HOUGH_GRADIENT, 1,minDist, param1=cmax, param2=thres, minRadius=minRadius, maxRadius=maxRadius)
dst=image.copy()
if circles is not None:
circles = np.uint16(np.around(circles))
for i in circles[0,:]:
# 원 둘레에 초록색 원 그리기
cv2.circle(dst,(i[0], i[1]), i[2], (0, 255, 0), 2)
# 원 중심점에 빨강색 원 그리기
cv2.circle(dst, (i[0], i[1]), 2, (0,0,255), 5)
cv2.imshow('img', dst)
cv2.imshow('img', image)
cv2.createTrackbar('minDist','img',1,200,on_trackbar)
cv2.setTrackbarPos('minDist','img',30)
cv2.createTrackbar('canny_max','img',0,500,on_trackbar)
cv2.setTrackbarPos('canny_max','img',240)
cv2.createTrackbar('thres','img',0,200,on_trackbar)
cv2.setTrackbarPos('thres','img',27)
cv2.createTrackbar('minRadius','img',0,200,on_trackbar)
cv2.setTrackbarPos('minRadius','img',20)
cv2.createTrackbar('maxRadius','img',0,200,on_trackbar)
cv2.setTrackbarPos('maxRadius','img',40)
cv2.waitKey()
cv2.destroyAllWindows()
https://docs.opencv.org/4.x/dd/d1a/group__imgproc__feature.html#ga47849c3be0d0406ad3ca45db65a25d2d
OpenCV: Feature Detection
param2Second method-specific parameter. In case of HOUGH_GRADIENT, it is the accumulator threshold for the circle centers at the detection stage. The smaller it is, the more false circles may be detected. Circles, corresponding to the larger accumulator va
docs.opencv.org
'Computer Vision > ImageProcessing' 카테고리의 다른 글
[OpenCV] Mask 설정 (0) | 2023.04.11 |
---|---|
[OpenCV] Template Matching (0) | 2023.04.10 |
[Python] 1채널 이미지를 3채널로 바꾸기 1Channel Image convert to 3 Channel Image (0) | 2022.12.21 |
BMP, JPG, JPEG, GIF, PNG 포맷의 특징 (0) | 2022.08.02 |
[OpenCV] install OpenCV on MacOS (0) | 2020.12.15 |